Entrust package provides a flexible way to add Role-based Permissions to your Laravel application.
This package creates four tables:
- Roles table: For storing role records
- Role_user table: For storing one-to-many relations between roles and users
- Permissions table: For storing permission records
- Permission_role table: For storing many-to-many relations between roles and permissions
Step 1: Install Entrust
open the composer.json in project and update the require object with entrust like this
OR
Open Terminal and run following command in root directory of your project
composer require zizaco/entrust:dev-master –no-update
Then Run
composer update
Step 2: Add Entrust Provider and Facades
Open up config/app.php, find the providers array and add the entrust provider:
Zizaco\Entrust\EntrustServiceProvider::class,
Find the aliases array and add the entrust facades:
‘Entrust’ => Zizaco\Entrust\EntrustFacade::class,
Then Run this command
php artisan vendor:publish
After this you will see a new file in config directory named entrust.php
If you want to use Middleware, You also need to add the following in the routeMiddleware array in app/Http/Kernel.php.
'role' => \Zizaco\Entrust\Middleware\EntrustRole::class,
'permission' => \Zizaco\Entrust\Middleware\EntrustPermission::class,
'ability' => \Zizaco\Entrust\Middleware\EntrustAbility::class,
Step 3: Now generate the Entrust migration:
php artisan entrust:migration
After the migration, four new tables will be present in the Database:
Roles, permissions, role_user, permission_role
Step 4: Create Models
1. Role Model
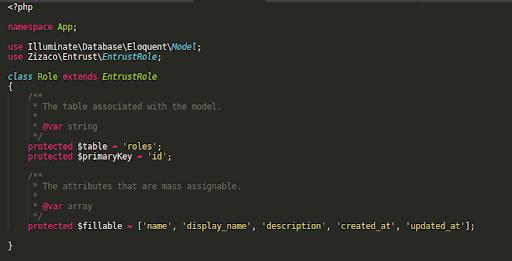
2. Permission Model
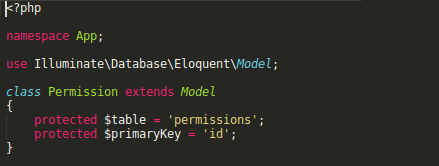
3. User Model
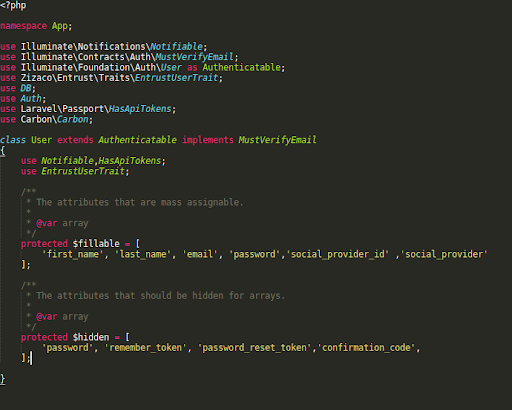
Now you can attach role to user at register time as per requirements like this:
Step 5 : Attaching roles and permissions
<?php
Route::get('/start', function()
{
$admin = new Role();
$admin->name = Admin;
$admin->save();
$customer = new Role();
$customer->name = ‘Customer’;
$customer->save();
$read = new Permission();
$read->name = 'can_read';
$read->display_name = 'Can Read Posts';
$read->save();
$edit = new Permission();
$edit->name = 'can_edit';
$edit->display_name = 'Can Edit Posts';
$edit->save();
$admin->attachPermission($read);
$customer->attachPermission($read);
$admin->attachPermission($edit);
$user1 = User::find(1);
$user2 = User::find(2);
$user1->attachRole($admin);
$user2->attachRole($customer);
return 'Woohoo!';
});
Now you can attach role to users at register time using this:
$user->roles()->attach(1);
To filter users according a specific role, you may use withRole() scope, for example to retrieve all admins:
$admins = User::withRole(‘admin’)->get();
Step 6 : Set Routes Role wise
Route::group(['middleware' => ['auth']], function()
{
Route::group(['prefix' => 'admin', 'middleware' => ['role:admin']], function() {
Route::get('/', 'AdminController@welcome');
});
Route::group(['prefix' => customer’, 'middleware' => ['role:customer']], function() {
Route::get('/', 'CustomerController@welcome');
});
});
Now you can access your project role wise.
To know more about how to implement Entrust in Laravel, please contact us.